Tickets kaufen
Tickets kaufen
Event- und Erlebnistickets
„Entdecken Sie außergewöhnliche Events und Abenteuer auf der Schmittenhöhe!"
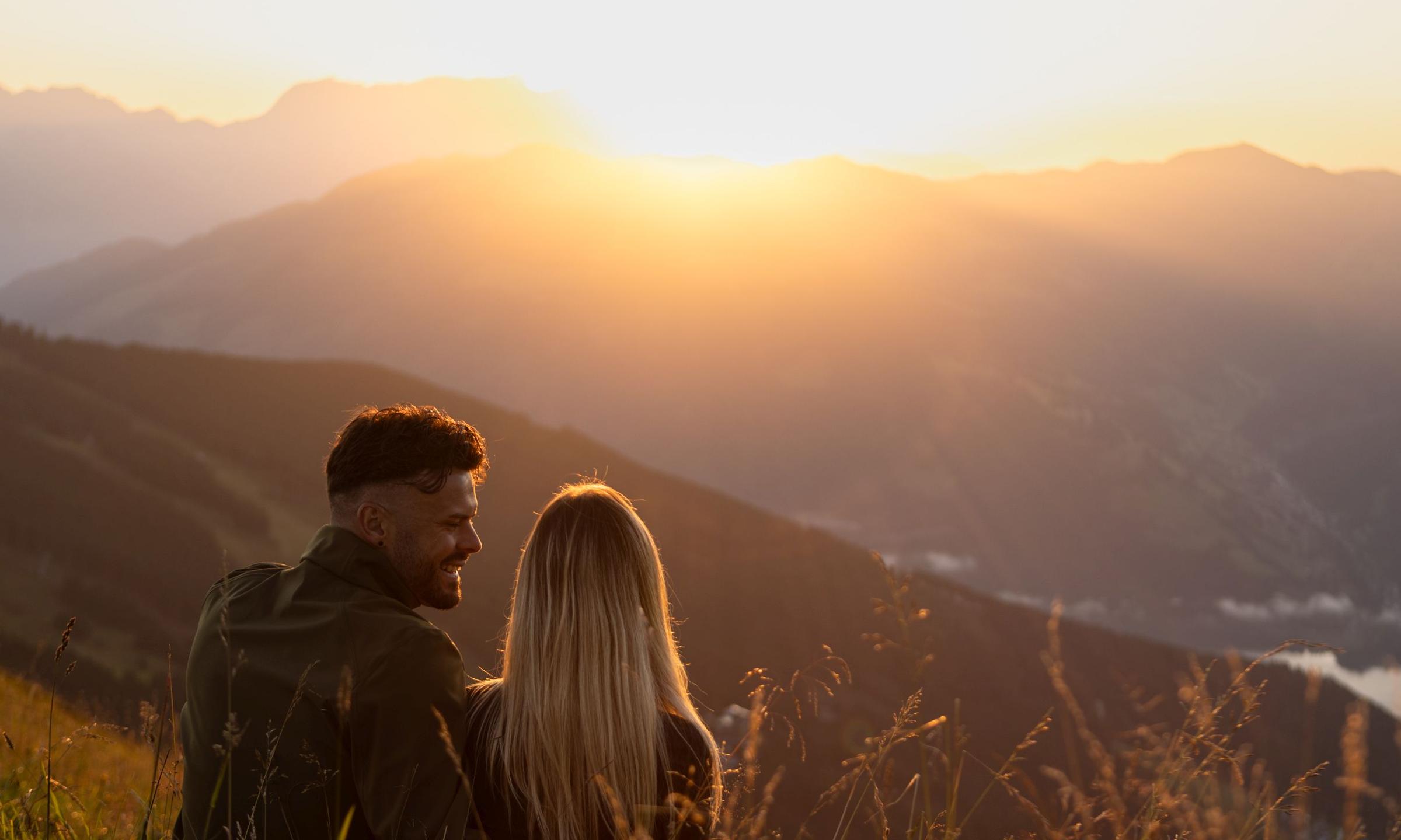
Hike'n'Brunch
26 Jul - 6 Sep
26. Juli 2025 | 05:00 Uhr30. August 2025 | 05:30 Uhr06. September 2025 | 05:45 Uhr
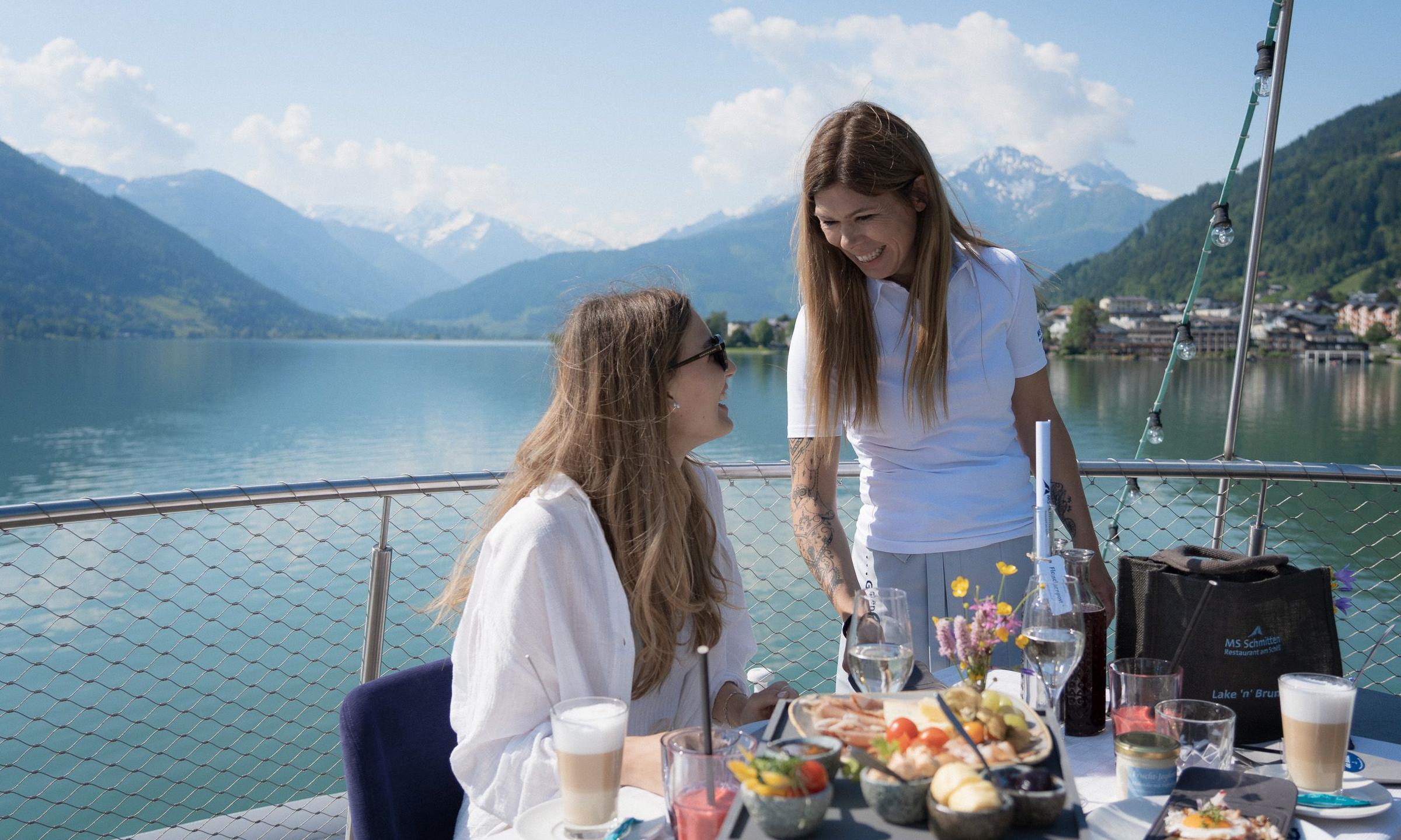
Lake'n'Brunch
20 Jul - 20 Sep | 09:00
14. Juni 2025 | 09:00-11:00 Uhr20. Juli 2025 | 09:00-11:00 Uhr23. August 2025 | 09:00-11:00 Uhr20. September 2025 | 09:00-11:00 Uhr
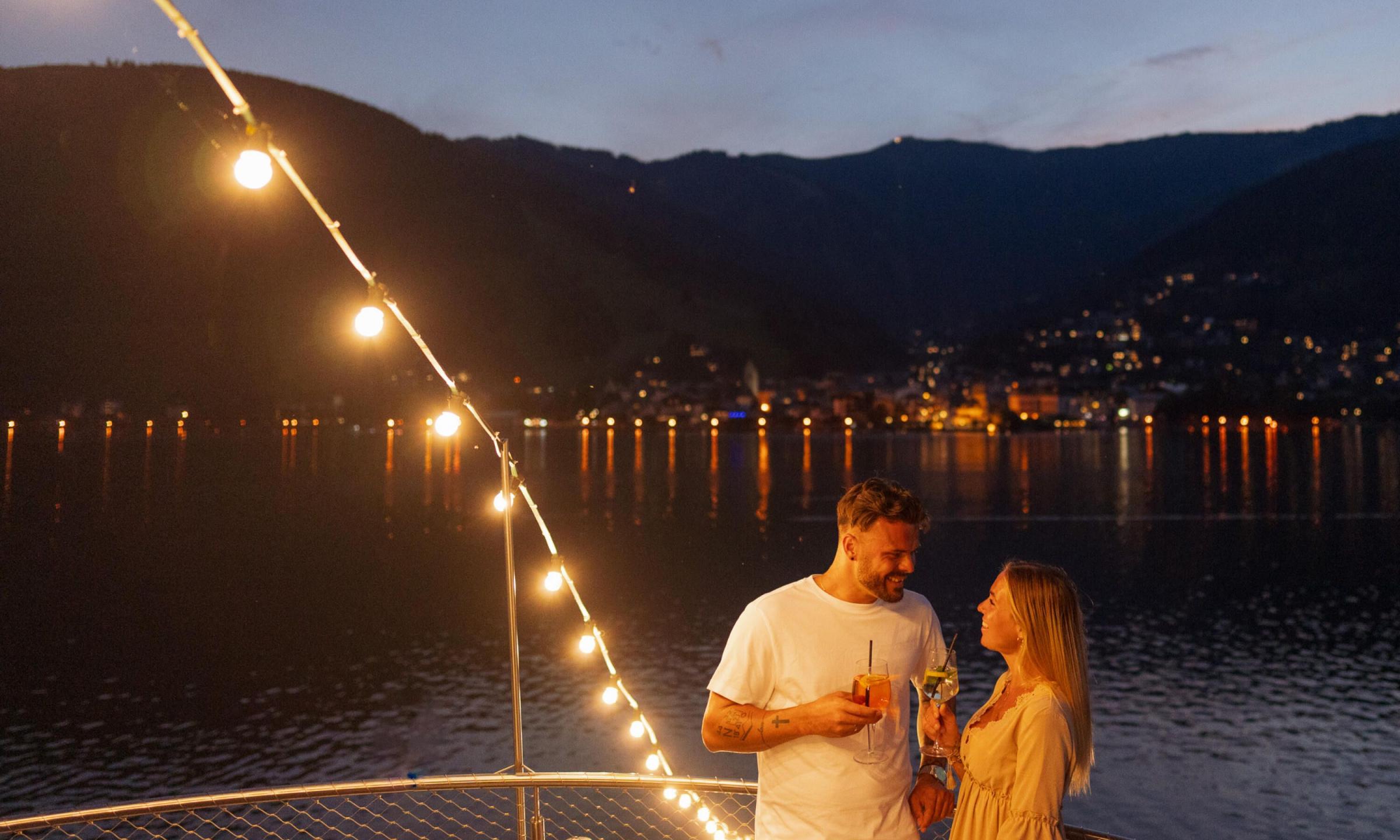
Feuerwerksrundfahrt Lounge
12 Jul - 2 Aug | 21:00
12. Juli 2025 | 21:00 Uhr02. August 2025 | 21:00 Uhr
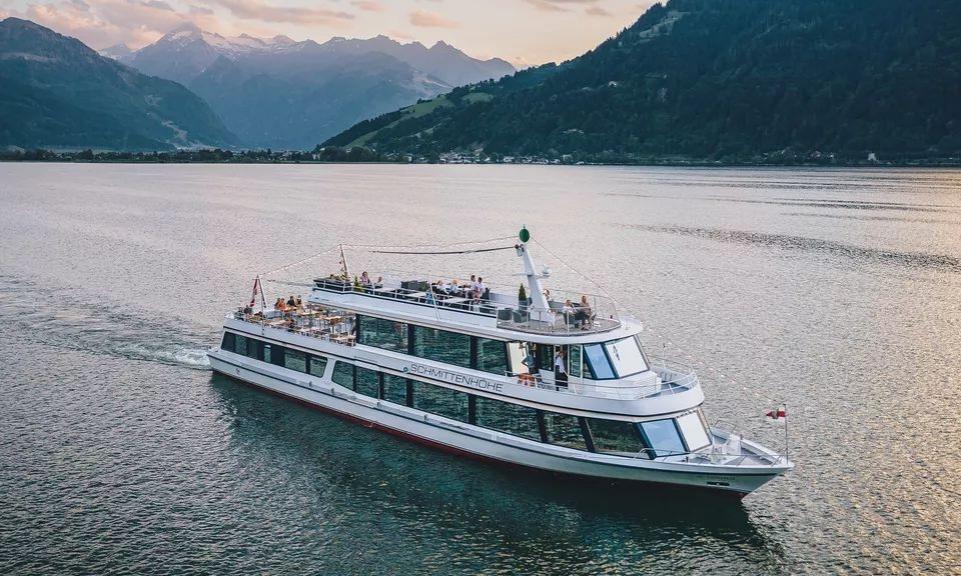
Abendrundfahrt
14 Jul - 25 Aug
Jeden Montagin der Zeit von 14.07. - 25.08.2025jeweils um 18:30 Uhr und 20:00 Uhr
Der Filter ergab keine Ergebnisse. Bitte versuchen Sie es mit einer anderen Kombination.